Code: Select all
import requests
ip = '192.168.0.1'
url = f'http://{ip}/logon.cgi'
data = {'username':'admin', 'password':'....', 'cpassword': '', 'logon':'Login'}
response = requests.post(url, data=data)
url = f'http://{ip}/PortStatisticsRpm.htm'
response = requests.get(url)
lines = response.text.split("\n")
pkts = [l for l in lines if l.startswith('pkts')][0]
pkts = pkts[6:-1].split(',')
for port in range(16):
print(f'port{port+1}_tx:{pkts[4*port+0]} ', end='')
print(f'port{port+1}_rx:{pkts[4*port+2]}', end='')
if(port<16): print(' ', end='')
Under Data Input Methods I have created a new tplink1016pe method with script "/usr/bin/python3 /path/to/tplink_sg1016pe.py", and 32 output fields (port1_rx, port1_tx, etc).port1_tx:5349846 port1_rx:3867312 port2_tx:2750447 port2_rx:1481102 port3_tx:6133576 port3_rx:3378887 port4_tx:4210717 port4_rx:1407855 port5_tx:1592126 port5_rx:750650 port6_tx:0 port6_rx:0 port7_tx:0 port7_rx:0 port8_tx:0 port8_rx:0 port9_tx:830444 port9_rx:182541 port10_tx:806663 port10_rx:8044 port11_tx:6102219 port11_rx:5181009 port12_tx:12247623 port12_rx:18245205 port13_tx:0 port13_rx:0 port14_tx:0 port14_rx:0 port15_tx:0 port15_rx:0 port16_tx:0 port16_rx:0
Under Data Source Templates I have created a data template based on the data input method tplink1016pe, with 32 data source items (port1_rx, port1_tx, etc, type DERIVE).
I'm stuck what the next step should be to get one graph for each port. Under Template, Graph, I have to create one graph template per port. That is an insane amount of clicking if I want 10 items on each graph:
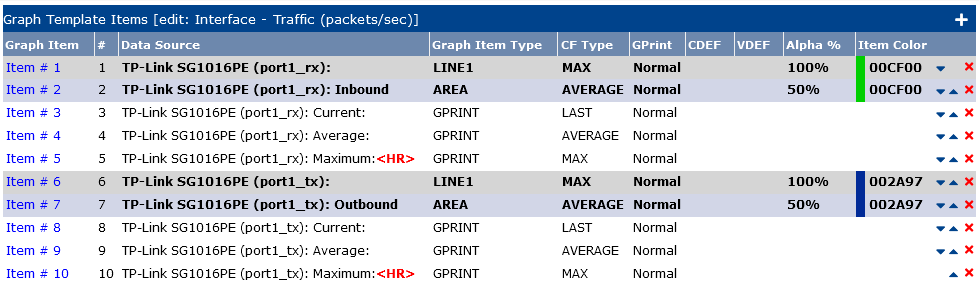
Is the solution to run the script once for each switch port, so the output fields are constant? Or is there another elegant solution?